若不进行处理,直接继续序列化添加对象进去。会在追加时继续写个头部的四个字节
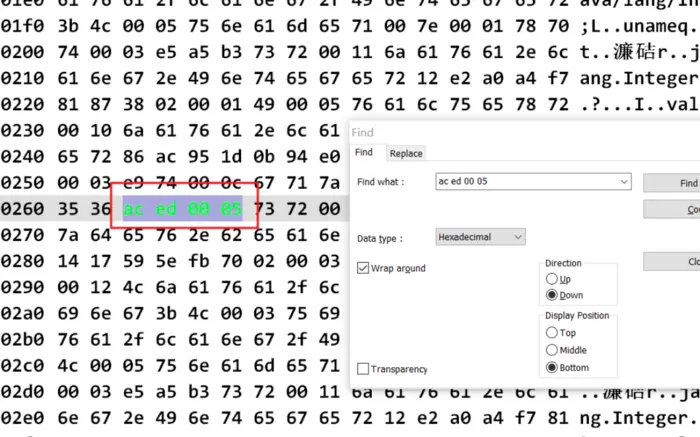
直接读取时,报错!
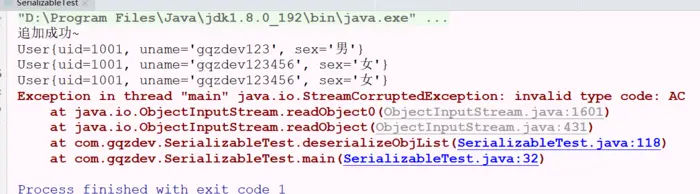

下面和我们手动去掉;
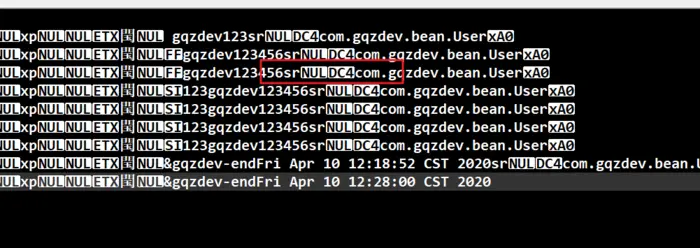
再次运行!
成功读取
package com.gqzdev;
import com.gqzdev.bean.User;
import java.io.*;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class SerializableTest implements Serializable {
public static void main(String[] args) throws IOException {
User user = new User(1001, "gqzdev-end"+new Date(), "女");
File file = new File("E:/ser");
List<User> objList = deserializeObjList(file);
System.out.println(objList.size());
for (User user1:objList) {
System.out.println(user1.toString());
}
}
public static void serialize(Object o,File file) throws IOException {
ObjectOutputStream stream =new ObjectOutputStream(new FileOutputStream(file));
try {
stream.writeObject(o);
stream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
public static void serializeAppend(Object obj,File file) throws IOException {
boolean isexist=false;
if (file.exists()){
isexist=true;
}
FileOutputStream fileOutputStream=new FileOutputStream(file,true);
ObjectOutputStream objectOutputStream = new ObjectOutputStream(fileOutputStream);
long pos = 0;
if (isexist) {
pos = fileOutputStream.getChannel().position() - 4;
fileOutputStream.getChannel().truncate(pos);
System.out.println("追加成功~");
}
objectOutputStream.writeObject(obj);
objectOutputStream.close();
}
public static Object deserialize(File file) throws IOException{
ObjectInputStream stream = new ObjectInputStream(new FileInputStream(file));
try {
Object o = stream.readObject();
stream.close();
return o;
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
return null;
}
public static List<User> deserializeObjList(File file) throws IOException{
List<User> list = new ArrayList<>();
FileInputStream fileInputStream = new FileInputStream(file);
ObjectInputStream stream = new ObjectInputStream(fileInputStream);
try {
while (fileInputStream.available()>0){
Object o = stream.readObject();
System.out.println(((User)o).toString());
list.add((User)o);
}
System.out.println("read-->"+list.size());
stream.close();
return list;
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
return null;
}
}