帮忙调试程序!栈的应用:行编辑器!该怎么处理
帮忙调试程序!栈的应用:行编辑器!
行编辑器的概念:接受用户从终端输入的程序或数据,并存入用户的数据区,用'#'表示退格符,用'@'表示退行符。
我们用的是严大妈的《数据结构》教材的。看它上面的为算法自己写的程序。
为什么第一个元素入不了栈?还是输出时候没有输出栈底的元素?
还有就是它书上怎么没有写,怎么输出栈的元素的?如果输出函数也是按栈顶到栈底的话,输出的结果是反的。比如我入栈是abcd#那么输出栈的元素就是cba(因为d被删除了。)。
------解决方案--------------------
指针参数传递二级指针试试,然后单步走一遍,代码粘贴过来居然没换行,真没劲一行一行的换了....
------解决方案--------------------
改为这个就可以。下一个为NULl,你就退出,所以少打印一个。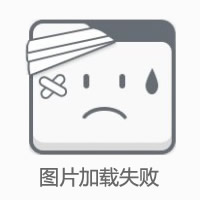
------解决方案--------------------
上面的两个空格 一个是你初始定义的 top->data = -1;一个是字符串结尾的'\0'
------解决方案--------------------
或者在你的基础上把这个改一改
行编辑器的概念:接受用户从终端输入的程序或数据,并存入用户的数据区,用'#'表示退格符,用'@'表示退行符。
我们用的是严大妈的《数据结构》教材的。看它上面的为算法自己写的程序。
为什么第一个元素入不了栈?还是输出时候没有输出栈底的元素?
还有就是它书上怎么没有写,怎么输出栈的元素的?如果输出函数也是按栈顶到栈底的话,输出的结果是反的。比如我入栈是abcd#那么输出栈的元素就是cba(因为d被删除了。)。
#include<stdio.h>
#include<stdlib.h>
#include<malloc.h>
//各种定义,才与教材的类型符合。
typedef int Status;
typedef char elemtype;
#define OK 1
#define ERROR -1
//数据的存储结构的定义。
typedef struct node{
elemtype data;
struct node *next;
}linkstack;
//各种函数的声明。
linkstack * Init_stack(void);
Status Push(linkstack *top,elemtype x);
bool Stack_Empty(linkstack *s);
Status print_stack(linkstack *s);
Status Pop(linkstack *top,elemtype &e);
Status DestroyStack(linkstack *top);
Status Edit(void);
int main()
{
Edit();
return 0;
}
//栈的初始化,带有头结点,不带有头结点的链式栈太难写了,也难操作。
linkstack * Init_stack(void)
{
linkstack *top;
top=(linkstack *)malloc(sizeof(linkstack) );
top->data=-1;
top->next=NULL;
return top;
}
//入栈
Status Push(linkstack *top,elemtype x)
{
linkstack * newnode,*temp;
newnode=(linkstack *)malloc(sizeof(linkstack) );
newnode->data=x;
newnode->next=top->next;
top->next=newnode;
return OK;
}
//出栈
Status Pop(linkstack *top,elemtype &e)
{
e=top->next->data;
linkstack * temp;
temp=top->next;
top->next=temp->next;
free(temp);
return OK;
}
//输出栈的元素
Status print_stack(linkstack *s)
{
for(linkstack *p=s;Stack_Empty(p)==false;p=p->next)
printf("%c",p->data);
printf("\n");
return OK;
}
//判断栈是否为空
bool Stack_Empty(linkstack *top)
{
if(top->next==NULL)
return true;
else
return false;
}
//销毁栈的函数
Status DestroyStack(linkstack *top)
{
elemtype temp;
while(top->next!=NULL)
Pop(top,temp);
return OK;
}
/*
行编辑器的函数,此函数完成调用各种函数的功能。
包括栈的初始化,入栈和出栈等功能,main函数就
只用来调用次函数就好了,所以我的main函数就只
有句语句啦!
*/
Status Edit(void)
{
elemtype ch;
linkstack *top;
top=Init_stack();
ch=getchar();
while(ch!=EOF&&ch!='\n')
{
switch(ch)
{
case '#':
Pop(top,ch);
break;
case '@':
DestroyStack(top);
break;
default:
Push(top,ch);
}
ch=getchar();
}
print_stack(top);
return OK;
}
编辑器
调试
栈
数据结构
------解决方案--------------------
指针参数传递二级指针试试,然后单步走一遍,代码粘贴过来居然没换行,真没劲一行一行的换了....
------解决方案--------------------
改为这个就可以。下一个为NULl,你就退出,所以少打印一个。
------解决方案--------------------
上面的两个空格 一个是你初始定义的 top->data = -1;一个是字符串结尾的'\0'
------解决方案--------------------
或者在你的基础上把这个改一改