Spring Boot (21) 使用Swagger2构建restful API
分类:
IT文章
•
2024-01-04 20:33:24
使用swagger可以与spring mvc程序配合组织出强大的restful api文档。它既可以减少我们创建文档的工作量,同时说明内容又整合入现实代码中,让维护文档和修改代码整合为一体,可以让我们在修改代码逻辑的同时方便的修改文档说明。另外swagger2也提供了强大的也卖弄测试功能来调试每个restful API。
首先搭建一个简单的restful api:
User.java
package com.example.bean;
public class User {
private Integer id;
private String name;
private Integer age;
public User() {
}
public User(Integer id, String name, Integer age) {
this.id = id;
this.name = name;
this.age = age;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
}
View Code
UserController.java
package com.example.controller;
import com.example.bean.User;
import com.example.dao.UserDao;
import org.springframework.web.bind.annotation.*;
import javax.annotation.Resource;
import java.util.*;
@RequestMapping("/users")
@RestController
public class UserController {
@Resource
private UserDao dao;
@GetMapping("/")
public List<User> getUserList() {
return dao.getAll();
}
@PostMapping("/")
public String postUser(@RequestBody User user) {
dao.addUser(user);
return "success";
}
@GetMapping("/{id}")
public User getUser(@PathVariable("id") Integer id) {
return dao.getUserById(id);
}
@PutMapping("/{id}")
public String putUser(@PathVariable("id") Integer id, @RequestBody User user) {
user.setId(id);
dao.updateUser(user);
return "success";
}
@DeleteMapping("/{id}")
public String deleteUser(@PathVariable("id") Integer id) {
dao.deleteUser(id);
return "success";
}
}
View Code
UserDao.java
package com.example.dao;
import com.example.bean.User;
import org.apache.ibatis.annotations.*;
import java.util.List;
@Mapper
public interface UserDao {
@Select("select * from user")
List<User> getAll();
@Select("select * from user where id = #{id}")
User getUserById(Integer id);
@Insert("insert into user (name,age) values (#{name},#{age})")
int addUser(User user);
@Delete("delete from user where id = #{id}")
int deleteUser(Integer id);
@Update("update user set name=#{name},age=#{age} where id = #{id}")
int updateUser(User user);
}
View Code
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>demo</name>
<description>Demo project for Spring Boot</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.2.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<!-- 引入mybatis -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>1.3.0</version>
</dependency>
<!--mysql数据库驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
View Code
application.yml
spring:
datasource:
url: jdbc:mysql://localhost:3306/david2018_db?characterEncoding=utf8
username: root
password: 1234
View Code
测试页面:
<html>
<head>
<title>Title</title>
<script src="http://libs.baidu.com/jquery/1.7.2/jquery.min.js"></script>
<script>
$(function(){
$("#btnAdd").click(function(){
var data = {
name:'david',
age:24
};
$.ajax({
url:'/users/',
type:"post",
data:JSON.stringify(data),
contentType:'application/json;charset=UTF-8',
dataType:"json",
success:function(data){
console.log(data)
}
});
});
$("#btnSearchAll").click(function () {
$.ajax({
url:'/users/',
type:'get',
contentType:'application/json;charset=UTF-8',
dataType:"json",
success:function(data){
console.log(data)
}
})
})
});
</script>
</head>
<body>
<button id="btnAdd">添加</button>
<button id="btnSearchAll">查询</button>
</body>
</html>
View Code
功能如下:
请求类型 |
URL |
功能说明 |
GET |
/users |
查询用户列表 |
POST |
/users |
创建一个用户 |
GET |
/users/id |
根据id查询一个用户 |
PUT |
/users/id |
根据id更新一个用户 |
DELETE |
/users/id |
根据id删除一个用户 |
下面来添加Swagger2依赖
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger2</artifactId>
<version>2.2.2</version>
</dependency>
<dependency>
<groupId>io.springfox</groupId>
<artifactId>springfox-swagger-ui</artifactId>
<version>2.2.2</version>
</dependency>
创建Swagger2配置类
在application.java同级创建Swagger2的配置类Swagger2.java
@Configuration
@EnableSwagger2
public class Swagger2 {
@Bean
public Docket createRestApi(){
return new Docket(DocumentationType.SWAGGER_2)
.apiInfo(apiInfo())
.select()
.apis(RequestHandlerSelectors.basePackage("com.example.controller"))
.paths(PathSelectors.any())
.build();
}
private ApiInfo apiInfo(){
return new ApiInfoBuilder()
.title("标题")
.description("详细描述")
.termsOfServiceUrl("http://www.david.com")
.contact("david")
.version("1.0")
.build();
}
}
@Configuration注解:让spring来加载该类配置。
@EnableSwagger2注解:启用Swagger2.
createRestApi函数创建Docket的Bean滞后,apiInfo()用来创建该Api的基本信息(这些基本信息会展示在文档页面中)。
select()函数返回一个ApiSelectorBuilder实例用来控制哪些接口暴露给Swagger来展现,本例扫描指定的Controller,会扫描所有Controller定义的API,并产生文档(除了被@ApiIgnore指定的请求)。
在controller中填写文档的详细描述: UserController.java
package com.example.controller;
import com.example.bean.User;
import com.example.dao.UserDao;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiImplicitParam;
import io.swagger.annotations.ApiImplicitParams;
import io.swagger.annotations.ApiOperation;
import org.springframework.web.bind.annotation.*;
import javax.annotation.Resource;
import java.util.*;
@RequestMapping("/users")
@RestController
public class UserController {
@Resource
private UserDao dao;
@GetMapping("/")
@ApiOperation(value="获取用户列表",notes = "")
public List<User> getUserList() {
return dao.getAll();
}
@PostMapping("/")
@ApiOperation(value = "创建用户",notes = "根据user对象创建用户")
@ApiImplicitParam(name="user",value="用户详细实体",required = true,dataType = "User")
public String postUser(@RequestBody User user) {
dao.addUser(user);
return "success";
}
@GetMapping("/{id}")
@ApiOperation(value = "获取用户详细信息",notes = "根据url中的id来获取用户信息")
@ApiImplicitParam(name = "id", value = "用户ID", required = true, dataType = "Integer")
public User getUser(@PathVariable("id") Integer id) {
return dao.getUserById(id);
}
@PutMapping("/{id}")
@ApiOperation(value="更新用户信息",notes = "根据url的id来查找信息,并根据传入的user修改信息")
@ApiImplicitParams({
@ApiImplicitParam(name = "id", value = "用户ID", required = true, dataType = "Long"),
@ApiImplicitParam(name = "user", value = "用户详细实体user", required = true, dataType = "User")
})
public String putUser(@PathVariable("id") Integer id, @RequestBody User user) {
user.setId(id);
dao.updateUser(user);
return "success";
}
@DeleteMapping("/{id}")
@ApiOperation(value="删除用户信息",notes = "根据url中的id来删除用户信息")
@ApiImplicitParam(name = "id", value = "用户ID", required = true, dataType = "Integer")
public String deleteUser(@PathVariable("id") Integer id) {
dao.deleteUser(id);
return "success";
}
}
启动spring boot程序,在浏览器输入:http://localhost:8080/swager-ui.html 就能看到restful API的页面,可以点开具体的API请求进行操作。
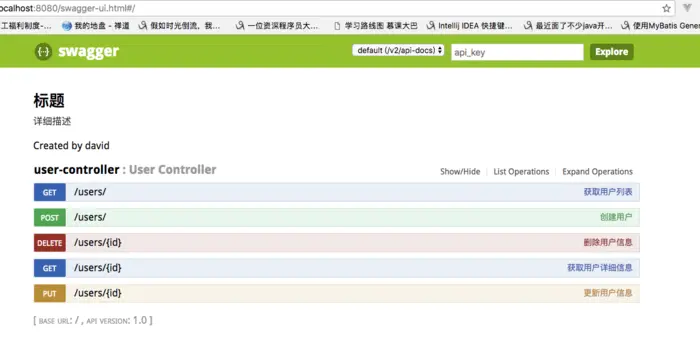