实验四 类的继承、派生和多态(1)
分类:
IT文章
•
2023-12-05 15:06:30
一、实验内容
1、车辆基本信息管理
基于Car类派生出ElectricCar类、派生类ElectricCar中新增数据成员Battery类对象。
#ifndef BATTERY_H
#define BATTERY_H
class Battery {
public:
Battery(int batterySize0=70);
int getSize();
private:
int batterySize;
};
#endif
battery.h
#ifndef CAR_H
#define CAR_H
#include<string>
#include<iostream>
using std::string;
using std::ostream;
class Car {
public:
Car(string maker0, string model0, int year0, int odometer0=0);
void updateOdometer(int odometerN);
friend ostream & operator<<(ostream &out, const Car &c);
private:
string maker;
string model;
int year;
int odometer;
};
#endif
car.h
#ifndef ELECTRIC_H
#define ELECTRIC_H
#include"battery.h"
#include"car.h"
class ElectricCar:virtual public Car{
public:
ElectricCar(string maker0,string model0,int year0,int odometer0=0);
friend ostream & operator<<(ostream &out, const ElectricCar &c);
private:
Battery battery;
};
#endif
electriccar.h
#include"battery.h"
#include<iostream>
using std::cout;
using std::endl;
Battery::Battery(int batterySize0 ):batterySize(batterySize0) {
}
int Battery::getSize(){
return batterySize;
}
battery.cpp
#include"car.h"
#include<iostream>
using std::cout;
using std::endl;
Car::Car(string maker0, string model0, int year0, int odometer0 ) :maker(maker0), model(model0), year(year0), odometer(odometer0) {
}
void Car::updateOdometer(int odometerN) {
if (odometerN < odometer) {
cout << "WRONG DATA!";
exit(0);
}
else odometer = odometerN;
}
ostream & operator<<(ostream &out, const Car &c) {
cout << "maker:" << c.maker << endl;
cout << "model:" << c.model << endl;
cout << "year:" << c.year << endl;
cout << "odometer:" << c.odometer << endl;
return out;
}
car.cpp
#include"electriccar.h"
#include<iostream>
using std::cout;
using std::endl;
ElectricCar::ElectricCar(string maker0, string model0, int year0, int odometer0 ):Car(maker0,model0,year0,odometer0) {
}
ostream & operator<<(ostream &out, const ElectricCar &c) {
Car a(c);
cout<<a<<endl;
Battery m=c.battery;
cout << "batterySize:" <<m.getSize()<<"-kWh"<< endl;
return out;
}
electriccar.cpp
#include <iostream>
using namespace std;
#include "car.h"
#include "electriccar.h"
int main() {
// 测试Car类
Car oldcar("Audi","a4",2016);
cout << "--------oldcar's info--------" << endl;
oldcar.updateOdometer(25000);
cout << oldcar << endl;
// 测试ElectricCar类
ElectricCar newcar("Tesla","model s",2016);
newcar.updateOdometer(2500);
cout << "
--------newcar's info--------
";
cout << newcar << endl;
system("pause");
return 0;
}
main.cpp
效果如下:
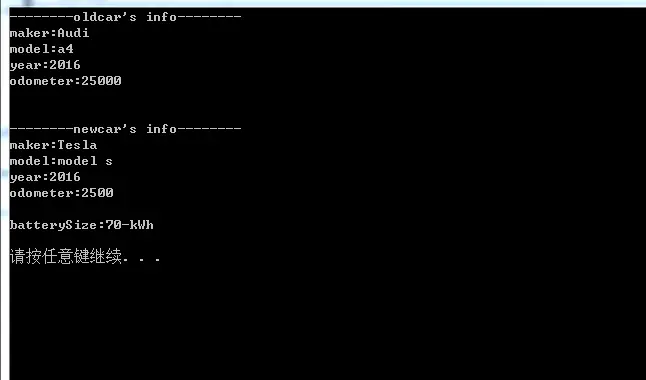
2、补足程序
重载运算符[]为一维动态整形数组类ArrayInt的成员函数,使得通过动态整形数组对象名和下标可以 访问对象中具体元素。
#ifndef ARRAY_INT_H
#define ARRAY_INT_H
class ArrayInt{
public:
ArrayInt(int n, int value=0);
~ArrayInt();
// 补足:将运算符[]重载为成员函数的声明
// ×××
int& operator[](int i);
void print();
private:
int *p;
int size;
};
#endif
arrayInt.h
#include "arrayInt.h"
#include <iostream>
#include <cstdlib>
using std::cout;
using std::endl;
ArrayInt::ArrayInt(int n, int value): size(n) {
p = new int[size];
if (p == nullptr) {
cout << "fail to mallocate memory" << endl;
exit(0);
}
for(int i=0; i<size; i++)
p[i] = value;
}
ArrayInt::~ArrayInt() {
delete[] p;
}
void ArrayInt::print() {
for(int i=0; i<size; i++)
cout << p[i] << " ";
cout << endl;
}
// 补足:将运算符[]重载为成员函数的实现
// ×××
int & ArrayInt::operator[](int i){
return p[i];
}
arrayInt.cpp
#include <iostream>
using namespace std;
#include "arrayInt.h"
int main() {
// 定义动态整型数组对象a,包含2个元素,初始值为0
ArrayInt a(2);
a.print();
// 定义动态整型数组对象b,包含3个元素,初始值为6
ArrayInt b(3, 6);
b.print();
// 通过对象名和下标方式访问并修改对象元素
b[0] = 2;
cout << b[0] << endl;
b.print();
system("pause");
return 0;
}
main.cpp
效果如下:
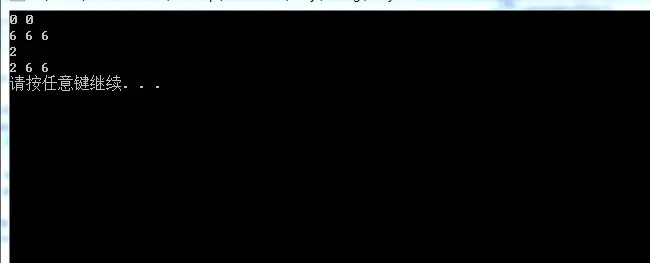
二、实验小结
虽然测试出结果,有几点还是不太懂:
- electriccar.cpp中,Car a(c); 我将ElectricCar类c赋值给Car类a,这是反复试探的结果,虽然意外成功,还是不太理解;
- 什么时候用&也不是很清楚。不对了,就加上&试试,偶尔可以猜对,却不是理解后的结果。
希望大佬不吝赐教,万分感激。
三、实验评论
- https://www.cnblogs.com/xuexinyu/p/10889782.html#4272146
- https://www.cnblogs.com/wjh1022/p/10890196.html#4272129
- https://www.cnblogs.com/jessi-wu1005/p/10889219.html#4272106