数独
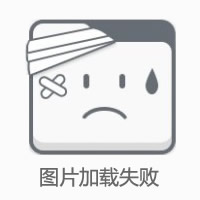
游戏规则
1.所有小方格填入数字1-9
2.每个数字在每行只能出现1次
3.每个数字在每列只能出现1次
4.每个数字在每宫只能出现1次
检查算法
//按行,按列,按宫
第6宫,序号 n = 5
坐标 x = n % 3 = 2
y = n / 3 = 1
每宫的起始格坐标 x0 = x * 3 = 6
y0 = y * 3 = 3
宫内小格坐标 x1 = x0 + i % 3
y1 = y0 + i / 3
坐标从宫内(0,0)开始
row = row - (row % 3)
col = col - (col % 3)
数独自动求解
const NOTASIGN = 0
function usedInCol(map,row,num){
for(let col = 0; col < map[0].length; col++){
if(map[row][col] === num){
return true
}
}
return false
}
function usedInRow(map,col,num){
for(let row = 0; row < map.length; row++){
if(map[row][col] === num){
return true
}
}
return false
}
function usedInBox(map,startRow,startCol,num){
for(let row = 0; row < 3; row++){
for(let col = 0; col < 3; col++){
if(map[startRow + row][startCol + col] === num){
return true
}
}
}
return false
}
function isSafe(map,row,col,num){
return (
!usedInRow(map,col,num)&&
!usedInCol(map,row,num)&&
!usedInBox(map,row - (row % 3),col - (col % 3),num)
)
}
function sudokuSolution(map){
let row = 0,
col = 0,
rowLen = map.length,
colLen = map[0].length,
checkBlankSpaces = false;
for(row = 0; row < rowLen; row++){
for(col = 0; col < colLen; col++){
if(map[row][col] === NOTASIGN){
checkBlankSpaces = true
break
}
}
if(checkBlankSpaces){
break
}
}
if(!checkBlankSpaces){
return true
}
for(let num =1; num<=9; num++){
if(isSafe(map,row,col,num)){
map[row][col] = num
if(sudokuSolution(map,row,col)){
return true
}
}
map[row][col] = NOTASIGN
}
return false
}
function sudokuSolver(map){
if(sudokuSolution(map)){
return map
}
return 'not result solution'
}
const sudokuGrid = [
[5, 3, 0, 0, 7, 0, 0, 0, 0],
[6, 0, 0, 1, 9, 5, 0, 0, 0],
[0, 9, 8, 0, 0, 0, 0, 6, 0],
[8, 0, 0, 0, 6, 0, 0, 0, 3],
[4, 0, 0, 8, 0, 3, 0, 0, 1],
[7, 0, 0, 0, 2, 0, 0, 0, 6],
[0, 6, 0, 0, 0, 0, 2, 8, 0],
[0, 0, 0, 4, 1, 9, 0, 0, 5],
[0, 0, 0, 0, 8, 0, 0, 7, 9]
];
console.log(sudokuSolver(sudokuGrid));