编写JDBC固定步骤
1、加载驱动
<dependencies>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
</dependencies>
2、连接数据库
String url = "jdbc:mysql://localhost:3306/jdbc?useUnicode=true&characterEncording=utf-8&useSSL=false";
String username="root";
String password="123456";
Connection connection = DriverManager.getConnection(url, username, password);
3、向数据库发送SQL对象Statement
Statement statement=connection.createStatement();
4、编写sql代码
String sql = "select * from users;";
5、执行sql(得到结果进行处理)
ResultSet resultSet = statement.executeQuery(sql);
while(resultSet.next()){
System.out.println("id:"+resultSet.getInt("id"));
System.out.println("名字:"+resultSet.getString("name"));
System.out.println("密码:"+resultSet.getString("password"));
System.out.println("邮箱:"+resultSet.getString("email"));
System.out.println("生日:"+resultSet.getString("birthday"));
System.out.println("===============================");
}
6、关闭连接,释放资源
resultSet.close();
statement.close();
connection.close();
代码
Statement代码
import java.sql.*;
public class test {
public static void main(String[] args) throws ClassNotFoundException, SQLException {
String url = "jdbc:mysql://localhost:3306/jdbc?useUnicode=true&characterEncording=utf-8&useSSL=false";
String username="root";
String password="123456";
Class.forName("com.mysql.jdbc.Driver");
Connection connection = DriverManager.getConnection(url, username, password);
Statement statement=connection.createStatement();
String sql = "select * from users;";
ResultSet resultSet = statement.executeQuery(sql);
while(resultSet.next()){
System.out.println("id:"+resultSet.getInt("id"));
System.out.println("名字:"+resultSet.getString("name"));
System.out.println("密码:"+resultSet.getString("password"));
System.out.println("邮箱:"+resultSet.getString("email"));
System.out.println("生日:"+resultSet.getString("birthday"));
System.out.println("===============================");
}
resultSet.close();
statement.close();
connection.close();
}
}
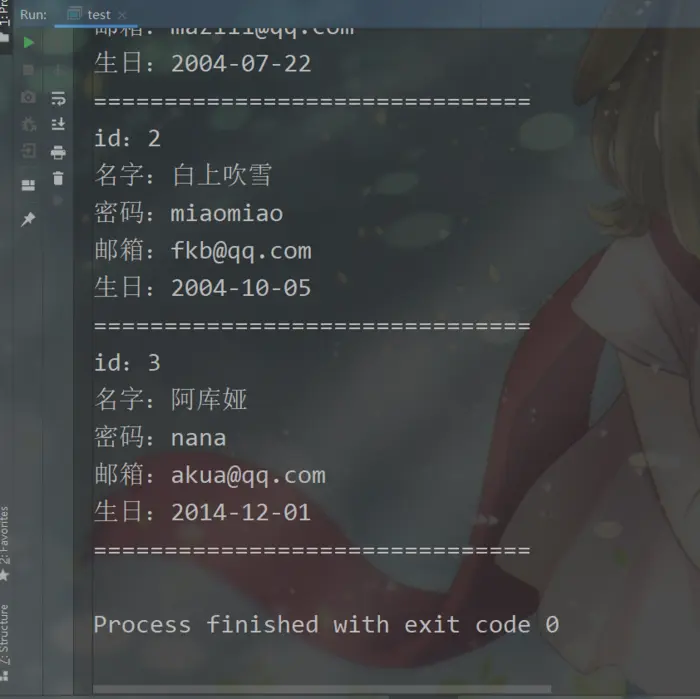
PreparedStatement 代码
import java.sql.*;
public class test2 {
public static void main(String[] args) throws ClassNotFoundException, SQLException {
String url = "jdbc:mysql://localhost:3306/jdbc?useUnicode=true&characterEncoding=utf-8&useSSL=false";
String username="root";
String password="123456";
Class.forName("com.mysql.jdbc.Driver");
Connection connection = DriverManager.getConnection(url, username, password);
String sql = "insert into jdbc.users(id, name, password, email, birthday) VALUE (?,?,?,?,?)";
PreparedStatement preparedStatement = connection.prepareStatement(sql);
preparedStatement.setInt(1, 4);
preparedStatement.setString(2, "鹿乃");
preparedStatement.setString(3, "kano");
preparedStatement.setString(4, "kano@qq.com");
preparedStatement.setString(5, "1995-12-24");
int i=preparedStatement.executeUpdate();
if(i>0){
System.out.println("插入成功");
}
preparedStatement.close();
connection.close();
}
}
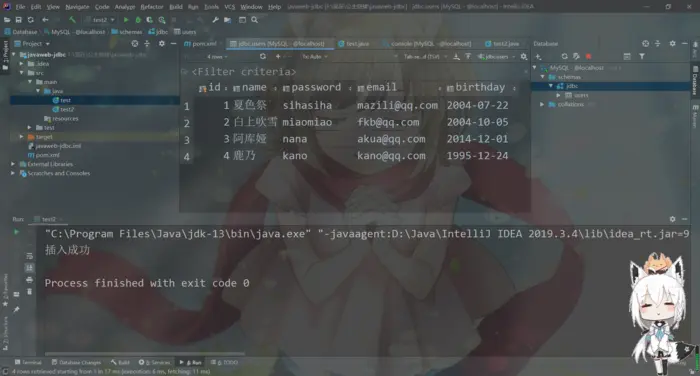