Spring Boot没有自己的文件上传下载技术。是体现于Sping MVC的文件上告上特下载技术,只不过在Spring Rod中做了更进一步的简化。
单文件上传
上传文件,必须将表单method设置为POST,并将enctype设置为 multipart/form-data。只有这样,浏览器才会把用户所选文件的二进制数据发送给服务器。Spring MVC在文件上传时,会将上传的文件映射为MultipartFile对象,并对MultipartFile对象进行文件的解析和保存。
MultipartFile接口中的几个常用方法:
byte[]getBytes():获取文件数据。
StringgetContentType():获取文件MIME类型,如application/pdf、image/pdf等。
InputStreamgetInputStream():获取文件流。
StringgetOriginalFileName():获取上传文件的原名称。
longgetSize():获取文件的字节大小,单位为byte。
booleanisEmpty():是否有上传的文件。
voidtransferTo(Filedest):将上传的文件保存到一个目标文件中。
多文件上传
多个文件的上传和单个文件的上传基本一致,只是在控制器里多一个遍历的步骤。
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.file</groupId>
<artifactId>File</artifactId>
<version>0.0.1-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.0.0.RELEASE</version>
<relativePath /> <!-- lookup parent from repository -->
</parent>
<properties>
<!-- 声明项目配置依赖编码格式为 utf-8 -->
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<project.reporting.outputEncoding>UTF-8</project.reporting.outputEncoding>
<fastjson.version>1.2.24</fastjson.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!--devtools热部署 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<optional>true</optional>
<scope>true</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
</project>
server.port=8008
spring.servlet.multipart.enabled=true
spring.servlet.multipart.max-file-size: 100MB
spring.servlet.multipart.max-file-size: 100MB
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-type" content="text/html; charset=UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1"/>
<title>单文件上传</title>
</head>
<body>
<form method="post" action="/file/upload" enctype="multipart/form-data">
<input type="file" name="file">
<input type="submit" value="提交">
</form>
<p>多文件上传</p>
<form method="POST" enctype="multipart/form-data" action="/file/uploads">
<!--添加multiple属性,可以按住ctrl多选文件-->
<p>文件:<input type="file" multiple name="file" /></p>
<p><input type="submit" value="上传" /></p>
</form>
</body>
</html>
package com.tszr.file.controller;
import java.io.File;
import java.util.List;
import java.util.UUID;
import javax.servlet.http.HttpServletRequest;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.multipart.MultipartHttpServletRequest;
@Controller
@RequestMapping("/file/")
public class FileController {
/* 单文件上传 */
@RequestMapping("upload")
@ResponseBody
public String upload(@RequestParam("file") MultipartFile file) {
// 获取原始名字
String fileName = file.getOriginalFilename();
// 获取后缀名
// String suffixName = fileName.substring(fileName.lastIndexOf("."));
// 文件保存路径
String filePath = "E:/file/";
// 文件重命名,防止重复
fileName = filePath + UUID.randomUUID() + fileName;
// 文件对象
File dest = new File(fileName);
// 判断路径是否存在,如果不存在则创建
if (!dest.getParentFile().exists()) {
dest.getParentFile().mkdirs();
}
try {
// 保存到服务器中
file.transferTo(dest);
return "上传成功";
} catch (Exception e) {
e.printStackTrace();
}
return "上传失败";
}
// 多文件上传
@PostMapping("/uploads")
@ResponseBody
public String handleFileUpload(HttpServletRequest request) {
List<MultipartFile> files = ((MultipartHttpServletRequest) request).getFiles("file");
MultipartFile file = null;
for (int i = 0; i < files.size(); ++i) {
file = files.get(i);
if (!file.isEmpty()) {
try {
// 获取原始名字
String fileName = file.getOriginalFilename();
// 文件保存路径
String filePath = "E:/files/";
// 文件重命名,防止重复
fileName = filePath + UUID.randomUUID() + fileName;
// 文件对象
File dest = new File(fileName);
// 判断路径是否存在,如果不存在则创建
if (!dest.getParentFile().exists()) {
dest.getParentFile().mkdirs();
}
// 保存到服务器中
file.transferTo(dest);
} catch (Exception e) {
e.printStackTrace();
return "上传失败";
}
} else {
return "上传失败";
}
}
return "上传成功";
}
}
package com.tszr.file;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SprtingApplication {
public static void main(String[] args) {
SpringApplication.run(SprtingApplication.class, args);
}
}
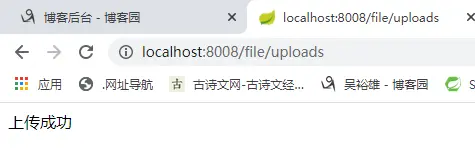
文件下载
文件下载时,我们可以直接输入url进行下载
package com.tszr.file.controller;
import java.io.File;
import java.io.FileInputStream;
import java.util.List;
import java.util.UUID;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.multipart.MultipartFile;
import org.springframework.web.multipart.MultipartHttpServletRequest;
@Controller
@RequestMapping("/file/")
public class FileController {
/* 单文件上传 */
@RequestMapping("upload")
@ResponseBody
public String upload(@RequestParam("file") MultipartFile file) {
// 获取原始名字
String fileName = file.getOriginalFilename();
// 获取后缀名
// String suffixName = fileName.substring(fileName.lastIndexOf("."));
// 文件保存路径
String filePath = "E:/file/";
// 文件重命名,防止重复
fileName = filePath + UUID.randomUUID() + fileName;
// 文件对象
File dest = new File(fileName);
// 判断路径是否存在,如果不存在则创建
if (!dest.getParentFile().exists()) {
dest.getParentFile().mkdirs();
}
try {
// 保存到服务器中
file.transferTo(dest);
return "上传成功";
} catch (Exception e) {
e.printStackTrace();
}
return "上传失败";
}
// 多文件上传
@PostMapping("/uploads")
@ResponseBody
public String handleFileUpload(HttpServletRequest request) {
List<MultipartFile> files = ((MultipartHttpServletRequest) request).getFiles("file");
MultipartFile file = null;
for (int i = 0; i < files.size(); ++i) {
file = files.get(i);
if (!file.isEmpty()) {
try {
// 获取原始名字
String fileName = file.getOriginalFilename();
// 文件保存路径
String filePath = "E:/files/";
// 文件重命名,防止重复
fileName = filePath + UUID.randomUUID() + fileName;
// 文件对象
File dest = new File(fileName);
// 判断路径是否存在,如果不存在则创建
if (!dest.getParentFile().exists()) {
dest.getParentFile().mkdirs();
}
// 保存到服务器中
file.transferTo(dest);
} catch (Exception e) {
e.printStackTrace();
return "上传失败";
}
} else {
return "上传失败";
}
}
return "上传成功";
}
// 文件下载
@RequestMapping("download")
public void download(HttpServletResponse response) throws Exception {
// 文件地址,真实环境是存放在数据库中的
String filename = "2bbeeca7-400e-4d12-bf1e-fc34c288ba4dsql.sql";
String filePath = "E:/file";
File file = new File(filePath + "/" + filename);
// 文件输入对象
FileInputStream fis = new FileInputStream(file);
// 设置相关格式
response.setContentType("application/force-download");
// 设置下载后的文件名以及header
response.setHeader("Content-Disposition", "attachment;fileName=" + filename);
}
}
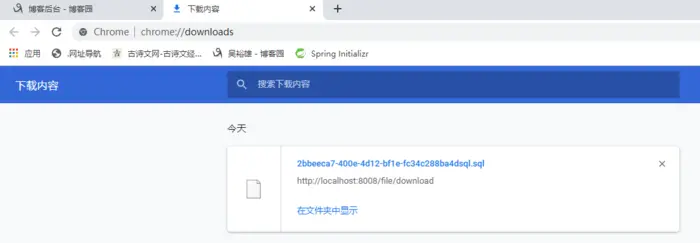