方格填数
题目描述
如下的10个格子
+--+--+--+
| | | |
+--+--+--+--+
| | | | |
+--+--+--+--+
| | | |
+--+--+--+
(如果显示有问题,也可以参看【图1.jpg】)
填入0~9的数字。要求:连续的两个数字不能相邻。
(左右、上下、对角都算相邻)
一共有多少种可能的填数方案?
请填写表示方案数目的整数。
注意:你提交的应该是一个整数,不要填写任何多余的内容或说明性文字。
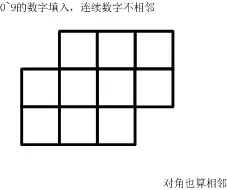
结果:1580
public class Main {
public static int count = 0;
public void swap(int[] A, int a, int b) {
int temp = A[a];
A[a] = A[b];
A[b] = temp;
}
public void dfs(int[] A, int step) {
if(step == A.length) {
if(check(A))
count++;
return;
} else {
for(int i = step;i < A.length;i++) {
swap(A, i, step);
dfs(A, step + 1);
swap(A, i, step);
}
}
return;
}
public boolean check(int[] A) {
if(Math.abs(A[0]-A[3]) != 1 && Math.abs(A[0]-A[1]) != 1 && Math.abs(A[0]-A[4]) != 1 && Math.abs(A[0]-A[5]) != 1) {
if(Math.abs(A[1]-A[4]) != 1 && Math.abs(A[1]-A[5]) != 1 && Math.abs(A[1]-A[2]) != 1 && Math.abs(A[1]-A[6]) != 1) {
if(Math.abs(A[2]-A[5]) != 1 && Math.abs(A[2]-A[6]) != 1) {
if(Math.abs(A[3]-A[4]) != 1 && Math.abs(A[3]-A[7]) != 1 && Math.abs(A[3]-A[8]) != 1) {
if(Math.abs(A[4]-A[5]) != 1 && Math.abs(A[4]-A[7]) != 1 && Math.abs(A[4]-A[8]) != 1 && Math.abs(A[4]-A[9]) != 1) {
if(Math.abs(A[5]-A[8]) != 1 && Math.abs(A[5]-A[9]) != 1 && Math.abs(A[5]-A[6]) != 1) {
if(Math.abs(A[6]-A[9]) != 1 && Math.abs(A[7]-A[8]) != 1) {
if(Math.abs(A[8]-A[9]) != 1)
return true;
}
}
}
}
}
}
}
return false;
}
public static void main(String[] args) {
Main test = new Main();
int[] A = {0,1,2,3,4,5,6,7,8,9};
test.dfs(A, 0);
System.out.println(count);
}
}