2014华为机考题-字符串压缩
2014华为机试题-字符串压缩
运行结果如下:

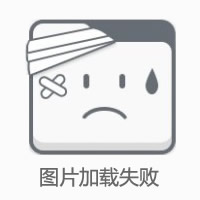

9月5日,华为2014校园招聘的机试题目
通过键盘输入一串小写字母(a~z)组成的字符串。请编写一个字符串压缩程序,将字符串中连续出席的重复字母进行压缩,并输出压缩后的字符串。
压缩规则:
1、仅压缩连续重复出现的字符。比如字符串"abcbc"由于无连续重复字符,压缩后的字符串还是"abcbc"。
2、压缩字段的格式为"字符重复的次数+字符"。例如:字符串"xxxyyyyyyz"压缩后就成为"3x6yz"。
要求实现函数:
void stringZip(const char *pInputStr, long lInputLen, char *pOutputStr);
输入pInputStr: 输入字符串lInputLen: 输入字符串长度
输出 pOutputStr: 输出字符串,空间已经开辟好,与输入字符串等长;
注意:只需要完成该函数功能算法,中间不需要有任何IO的输入输出
示例
输入:“cccddecc” 输出:“3c2de2c”
输入:“adef” 输出:“adef”
输入:“pppppppp” 输出:“8p”
1、仅压缩连续重复出现的字符。比如字符串"abcbc"由于无连续重复字符,压缩后的字符串还是"abcbc"。
2、压缩字段的格式为"字符重复的次数+字符"。例如:字符串"xxxyyyyyyz"压缩后就成为"3x6yz"。
要求实现函数:
void stringZip(const char *pInputStr, long lInputLen, char *pOutputStr);
输入pInputStr: 输入字符串lInputLen: 输入字符串长度
输出 pOutputStr: 输出字符串,空间已经开辟好,与输入字符串等长;
注意:只需要完成该函数功能算法,中间不需要有任何IO的输入输出
示例
输入:“cccddecc” 输出:“3c2de2c”
输入:“adef” 输出:“adef”
输入:“pppppppp” 输出:“8p”
用VC6.0写了个程序调试了下
代码如下:
#include <stdio.h> #include <stdlib.h> #define MAXCHAR 5000 //最长处理5000个字符 void stringZip(const char *pInputStr, long lInputLen, char *pOutputStr); int main() { char ch; char input[MAXCHAR]; char output[MAXCHAR]; int i = 0; printf("Input string: "); while((ch = getchar()) != '\n') { input[i++] = ch; } input[i] = '\0'; stringZip(input, i, output); printf("Before string zip method: %s\n", input); printf("After string zip method: %s\n", output); return 0; } void stringZip(const char *pInputStr, long lInputLen, char *pOutputStr) { int num = 1; int j = 0; for(int i = 1; i <= lInputLen; i++) { if(*(pInputStr + i) == *(pInputStr + i - 1)) { num++; } else { if(num == 1) { *(pOutputStr + j) = *(pInputStr + i - 1); j++; } else if(num < 10 && num > 1) { *(pOutputStr + j) = '0' + num; j++; *(pOutputStr + j) = *(pInputStr + i - 1); j++; } else if(num > 9 && num < 100) { *(pOutputStr + j) = '0' + (num / 10); j++; *(pOutputStr + j) = '0' + (num % 10); j++; *(pOutputStr + j) = *(pInputStr + i - 1); j++; } else if(num >= 100) { *(pOutputStr + j) = '?'; j++; *(pOutputStr + j) = *(pInputStr + i - 1); j++; } num = 1; } } *(pOutputStr + j) = '\0'; }
运行结果如下: