android惯用控件(三)- ProgressBar、ListView
android常用控件(三)- ProgressBar、ListView
Android常用控件(三)- ProgressBar、ListView
一、 ProgressBar(进度条)的使用
示例1:一个应用程序有2个ProgressBar,让进度条显示进度。
开发步骤:
1、 新建一个android项目
2、 在main.xml布局文件中先添加1个ProgressBar控件(firstProgressBar),设置这个进度条是以水平方式展示的,然后再设置这个控件为不显示(这里暂时不显示,我们在后面的程序中写代码将这个控件设置为显示)
3、 在main.xml布局文件中再添加1个ProgressBar控件(secondProgressBar),设置该标签的显示样式为默认的(是一个转动的圆圈),然后再同样设置这个控件暂时为不显示
4、 在main.xml布局文件中再添加一个Button控件
5、 在Activity中编写代码,先获得这2个ProgressBar和Button对象
6、 然后编写一个监听器,设置进度条的进度,当每点击一次Button,进度则增加10
7、 将监听器绑定到Button对象上
下图为main.xml布局文件的片段:

Activity的代码在这里就没有贴出来了,项目源码已经上传,有需要的可以下载。
最后项目实现的效果为:
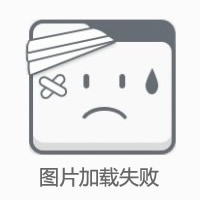
二、 ListView的使用
ListView的使用相对于之前使用到的一些控件而言要复杂一点,在这里呢,同样是通过一个示例来讲解,但是在这过程中,可能有些理解没有很准确或者注释的不是准确的地方,到时候有什么问题还请大家多多指点指点哦~
(如果各位E文比较好的可以去官网查看更详细的说明)
示例2:一个应用程序有一个ListView,显示三行信息。
最后项目实现的效果为:
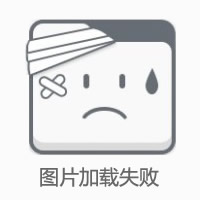
开发步骤:
1、 新建一个Android应用程序
2、 在布局文件中再添加一个LinearLayout(应用程序新建的时候默认的布局文件里就已经有了一个LinearLayout,现在再添加一个),设置这个LinearLayout的一些属性。
3、 在LinearLayout中添加一个ListView,并设置一些属性。
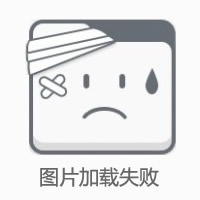
4、 新建一个布局文件,这个布局文件呢,是用来布局和显示ListView里面的内容的(我是这样理解的,不知道大家能不能理解哈)。先在这个布局文件中添加一个LinearLayout,然后在LinearLayout中添加两个TextView,表示显示两列数据(如果需要显示多列数据的话当然就是添加多个TextView了)。
5、 编写Activity
a) 首先要注意的是,这个Activity他继承的不是Activity,而是ListActivity。
b) 创建一个ArrayList,里面存放的是HashMap,而HashMap的键值对都是String类型。
c) 分别往3个HashMap中存储值
d) 将3个HashMap添加到ArrayList中
e) 创建适配器
f) 绑定到适配器
g) 编写行的点击事件
项目源码已经上传到附件,有需要的可下载。
Android常用控件(三)- ProgressBar、ListView
一、 ProgressBar(进度条)的使用
示例1:一个应用程序有2个ProgressBar,让进度条显示进度。
开发步骤:
1、 新建一个android项目
2、 在main.xml布局文件中先添加1个ProgressBar控件(firstProgressBar),设置这个进度条是以水平方式展示的,然后再设置这个控件为不显示(这里暂时不显示,我们在后面的程序中写代码将这个控件设置为显示)
3、 在main.xml布局文件中再添加1个ProgressBar控件(secondProgressBar),设置该标签的显示样式为默认的(是一个转动的圆圈),然后再同样设置这个控件暂时为不显示
4、 在main.xml布局文件中再添加一个Button控件
5、 在Activity中编写代码,先获得这2个ProgressBar和Button对象
6、 然后编写一个监听器,设置进度条的进度,当每点击一次Button,进度则增加10
7、 将监听器绑定到Button对象上
下图为main.xml布局文件的片段:
Activity的代码在这里就没有贴出来了,项目源码已经上传,有需要的可以下载。
最后项目实现的效果为:
二、 ListView的使用
ListView的使用相对于之前使用到的一些控件而言要复杂一点,在这里呢,同样是通过一个示例来讲解,但是在这过程中,可能有些理解没有很准确或者注释的不是准确的地方,到时候有什么问题还请大家多多指点指点哦~
(如果各位E文比较好的可以去官网查看更详细的说明)
示例2:一个应用程序有一个ListView,显示三行信息。
最后项目实现的效果为:
开发步骤:
1、 新建一个Android应用程序
2、 在布局文件中再添加一个LinearLayout(应用程序新建的时候默认的布局文件里就已经有了一个LinearLayout,现在再添加一个),设置这个LinearLayout的一些属性。
3、 在LinearLayout中添加一个ListView,并设置一些属性。
4、 新建一个布局文件,这个布局文件呢,是用来布局和显示ListView里面的内容的(我是这样理解的,不知道大家能不能理解哈)。先在这个布局文件中添加一个LinearLayout,然后在LinearLayout中添加两个TextView,表示显示两列数据(如果需要显示多列数据的话当然就是添加多个TextView了)。
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="horizontal" android:layout_width="fill_parent" android:layout_height="fill_parent" android:paddingTop="1dip" android:paddingBottom="1dip" android:paddingLeft="10dip" android:paddingRight="10dip" > <TextView android:id="@+id/user_name" android:layout_width="180dip" android:layout_height="30dip" android:textSize="10pt" android:singleLine="true" /> <TextView android:id="@+id/user_ip" android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="10pt" android:gravity="right" /> </LinearLayout>
5、 编写Activity
a) 首先要注意的是,这个Activity他继承的不是Activity,而是ListActivity。
b) 创建一个ArrayList,里面存放的是HashMap,而HashMap的键值对都是String类型。
c) 分别往3个HashMap中存储值
d) 将3个HashMap添加到ArrayList中
e) 创建适配器
f) 绑定到适配器
g) 编写行的点击事件
package android.listview; import java.util.ArrayList; import java.util.HashMap; import android.app.ListActivity; import android.os.Bundle; import android.view.View; import android.widget.ListView; import android.widget.SimpleAdapter; //注意:这里继承的是ListActivity,而不是Activity public class ListViewTest extends ListActivity { /** Called when the activity is first created. */ @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); // 创建一个ArrayList,ArrayList里面存放的是HashMap,而HashMap的键值对都是String类型 ArrayList<HashMap<String, String>> list = new ArrayList<HashMap<String, String>>(); HashMap<String, String> map1 = new HashMap<String, String>(); HashMap<String, String> map2 = new HashMap<String, String>(); HashMap<String, String> map3 = new HashMap<String, String>(); // 分别往3个HashMap中存储值 map1.put("user_name", "zhangsan"); map1.put("user_ip", "192.168.0.1"); map2.put("user_name", "lisi"); map2.put("user_ip", "192.168.0.2"); map3.put("user_name", "wangwu"); map3.put("user_ip", "192.168.0.3"); // 将3个HashMap添加到ArrayList中 list.add(map1); list.add(map2); list.add(map3); //创建适配器 //第一个参数Content:上下文 //第二个参数List<? extends Map<String, ?>>:ArrayList对象,ArrayList里面存放的是HashMap,而HashMap的键值对都是String类型 //第三个参数int resource:内容显示的布局文件 //第四个参数String[] from:被添加到ArrayList中的HashMap中key的名称,要显示的列 //第五个参数int[] to:内容显示的布局文件中,显示内容的控件id SimpleAdapter listAdapter = new SimpleAdapter(this, list, R.layout.user, new String[] { "user_name", "user_ip" }, new int[] { R.id.user_name, R.id.user_ip }); //绑定到适配器。 setListAdapter(listAdapter); } /** * 列表当中一行的点击事件 * ListView:ListView对象本身 * View:被选中的那一行的View对象 * position:被选中的那一行的位置 * id:被选中的那一行的id */ @Override protected void onListItemClick(ListView l, View v, int position, long id) { // TODO Auto-generated method stub super.onListItemClick(l, v, position, id); //打印出被选中的那一行的位置和id,计数都是从0开始 System.out.println("-------------"+position); System.out.println("-------------"+id); } }
项目源码已经上传到附件,有需要的可下载。
1 楼
byandby
2010-12-02
BB 加油 。。
2 楼
sunjiesh
2011-12-29
照着例子写了一下,发现报java.lang.RuntimeException: Your content must have a ListView whose id attribute is 'android.R.id.list'异常,后来查了一下,发现只要把setContentView(R.layout.main);注释后就可以正常了。
参考:http://*.com/questions/5086467/why-must-i-have-a-listview
参考:http://*.com/questions/5086467/why-must-i-have-a-listview
3 楼
byandby
2011-12-29
sunjiesh 写道
照着例子写了一下,发现报java.lang.RuntimeException: Your content must have a ListView whose id attribute is 'android.R.id.list'异常,后来查了一下,发现只要把setContentView(R.layout.main);注释后就可以正常了。
参考:http://*.com/questions/5086467/why-must-i-have-a-listview
参考:http://*.com/questions/5086467/why-must-i-have-a-listview